Saving and Loading Chunks
SimpleMiner (Minecraft-Like)
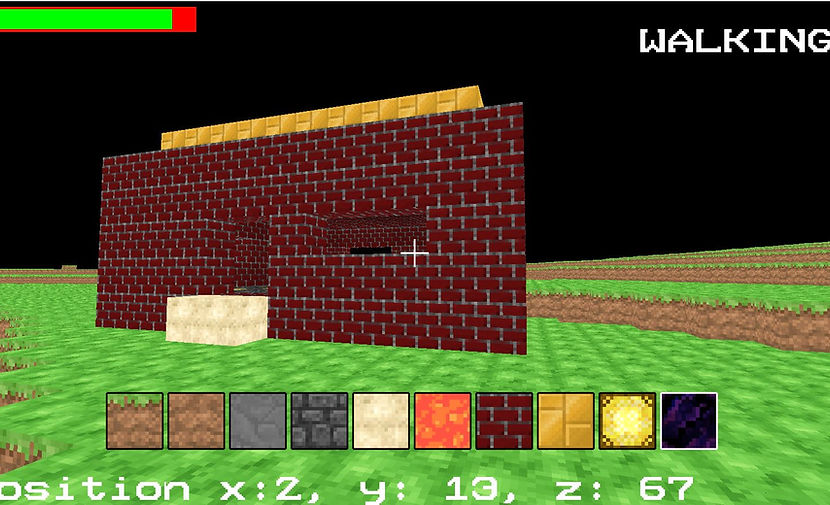
Technology
-
Visual Studio (2013/2015)
-
C++
-
GLSL (OpenGL)
-
-
Photoshop
​
Genre SinglePlayer, 3D, Minecraft-Like
​
Platform PC (Windows)
​
Development Time 2 Months
Resources Used
Table of Contents
Game Summary
This game was aimed towards making something similar to Minecraft's Creative mode. As such the core functionallity in this game is aimed towards creating and destroying blocks, to build whatever the player wants. It is worth noting that the movement controls are different from the norm, in that instead of WASD, it uses ESDF; this is due to a special request of my instructor at the time of creating this project.
The big things that were worked on in this project are: Placing and Destroying blocks, Procedural World Generation through Perlin Noise (including Saving and Loading chunks), Perlin Worms for the tunnels, Some basic 3D collision between box colliders, Lighting, and a Jetpack.
Controls
E - Move forward
D - Move backwards
S - Straif Left (No Momentum)
F - Straif Right (No Momentum)
Left Click - Destroy Blocks
Right Click - Place blocks
Mouse Wheel - Scroll through Block Selection
W - Select previous block type
R - Select next block type
0 through 9 - Select the specific corresponding block in the selection
P - Movement modes (No Clip, Flying, Walking) (Walking is default)
B - Toggle Debug ViewModel (AABB3 Lines)
F5 - Cycle through Camera modes (First Person, Third Person, and Fixed Angle)
SPACE (When Walking) - Jump; Hold for Jetpack
SPACE (When in No Clip or Flying) - Move up
Z (When in No Clip or Flying) - Move down
SHIFT (hold) - Move 8 times as fast
​
How to erase save data:
Go into the folder "Data/save01/", if in windows, press the keys Ctrl + A, and then delete all of the files. Removing the save01 folder will make it to where saving the chunks is impossible.
Generating the Chunks (Procedural World Generation through Perlin Noise)
-
World Generation uses Perlin Worms and Perlin Noise.
-
Perlin Noise is a type of controlled random generation, creates a noise map that can gradually transition based on position in the map.
-
Can use that Perlin Noise map data to generate heights of the column, block placement, and generally create mountains, hills or lakes.
-
Perlin Worms use Perlin Noise to generate chains of lines towards given points, and as an example can be used to generate Caverns and Caves.
-
Perlin Noise is first used to generate scores along a grid near a chunk being generated, then after a certain score is found, thats above a certain defined score, that area is a cave start area.
-
Afterwards, Perlin Noise is used to generate the number of points that the cave will have, the starting rotation for the cave to follow, and alterations to the Perlin Caves' rotation.
-
Additionally, Perlin Noise can be used to generate the cave's radius at a given point.
-
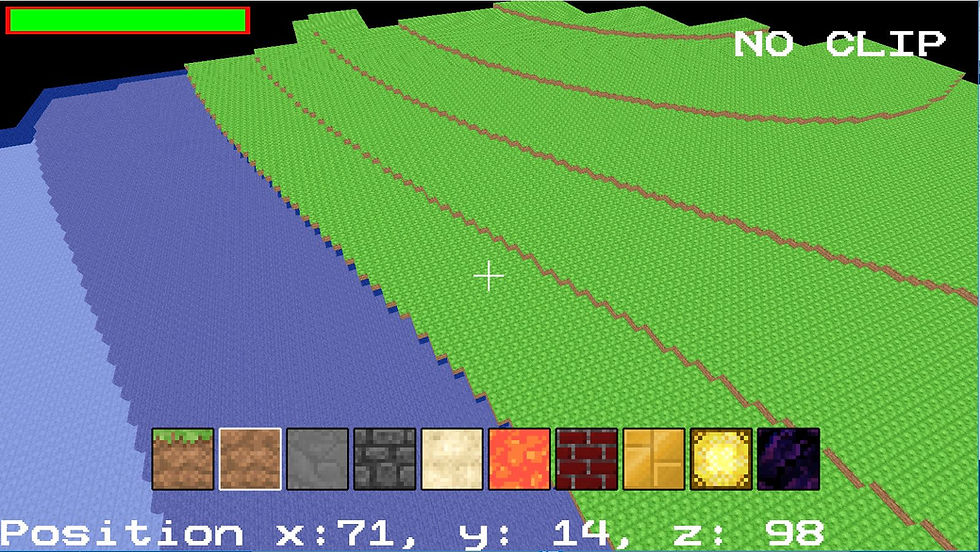
Here we are viewing some chunks which were generated using perlin noise. You should be able to see that the ground is slowly rising up out of a lake like area, and into hills.
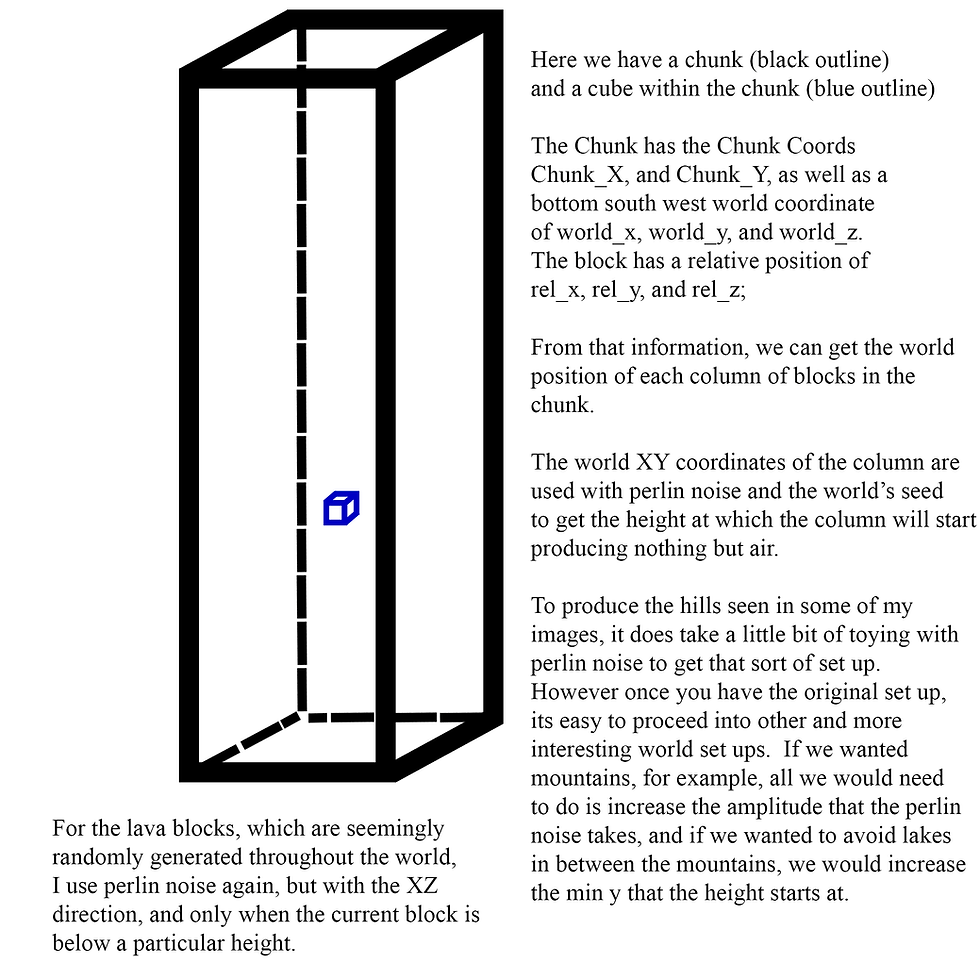
This is an explanation for how perlin noise is used in simple miner's chunk generation.
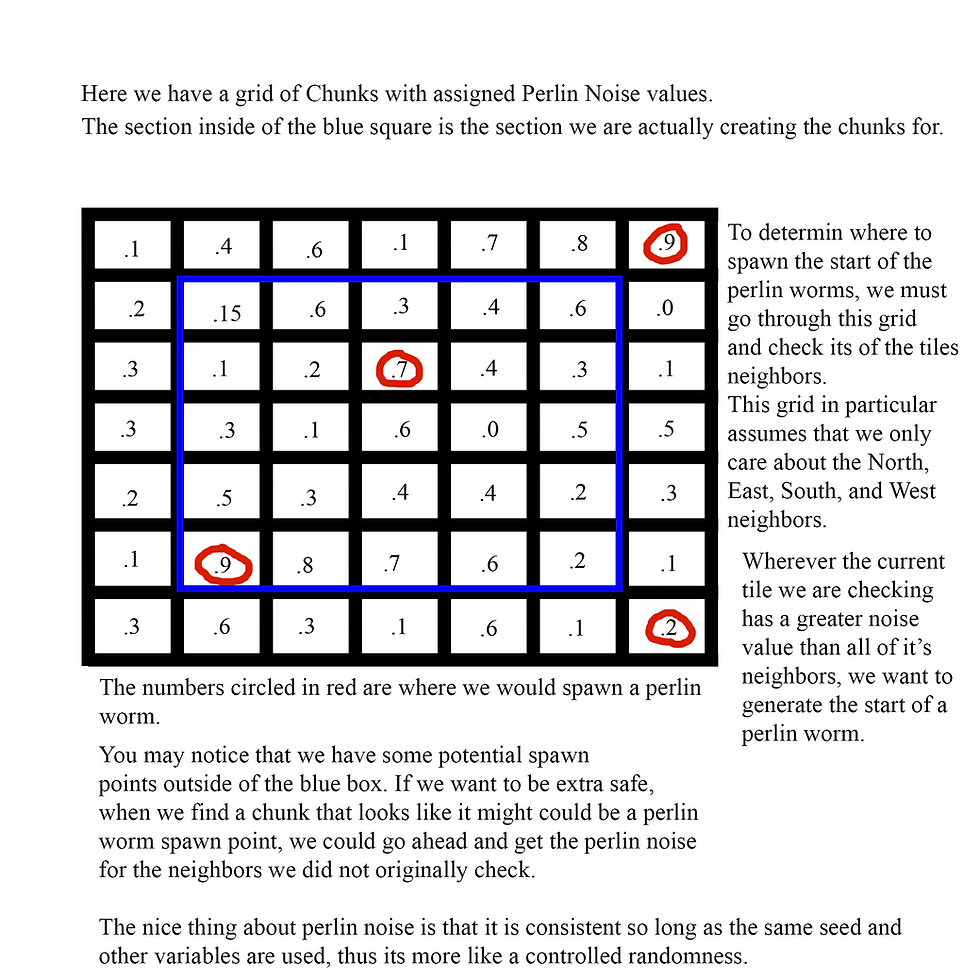
This is an explanation for how to generate where to start spawning Perlin Worms. Once you have the Perlin worms at their spawn location, you just use the chunk coordinates to generate how many cave points the worm will have, as well as the starting direction the worm will go. Each new cave point will use perlin noise to generate the radius of the cave at that point, as well as how much to tweak the direction that the worm is currently going. The worm turns almost every block it touches into air.
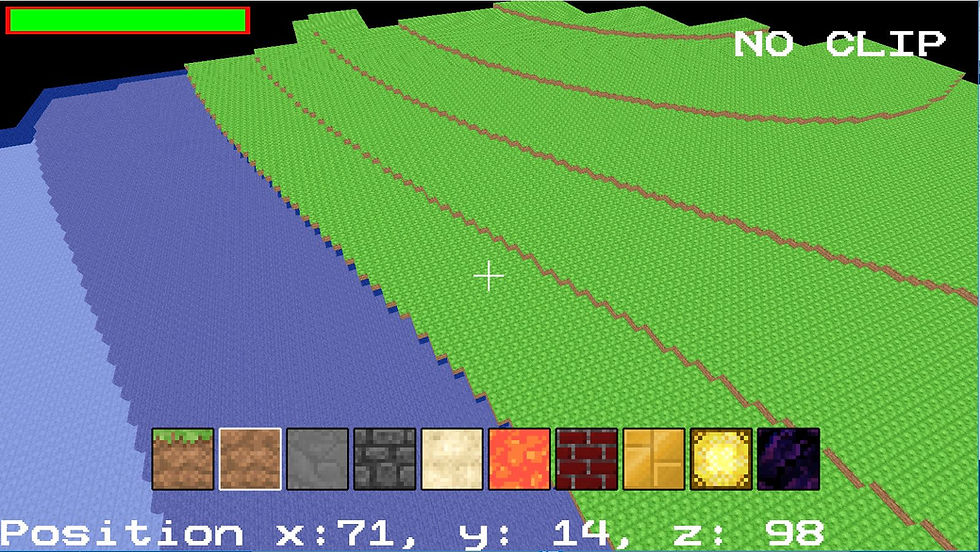
Here we are viewing some chunks which were generated using perlin noise. You should be able to see that the ground is slowly rising up out of a lake like area, and into hills.
Chunk Generation Code within Chunk class
Cave Generation Code within the Cave Class.
Summarized Bullet Points:
-
Chunks that are generated/Loaded use a radius out from the player to determine should be generated/loaded
-
There is an even bigger radius for determining should unload and save off chunks.
-
Priority between unloading and loading is given to loading chunks first.
​
Generating chunks occurs whenever the player gets close enough to a chunk they've never encountered before. But what about saving and reloading previously loaded chunks? When generating chunks, there is a radius about the player that would normally be cared about for when to generate chunks. The same radius is used with loading chunks. As for saving off chunks and unloading them, those radius-es are bigger than the radius used for generating/loading chunks. The priority is given first to loading chunks, then to generating new chunks, then to saving off and deleting chunks. This is so as if the player needs to run back and forth, it wont of removed the chunks that would of needed to be loaded off again immediately. The chunks that get loaded/generated first are those that are closest to the player, within the load/generation radius.
Chunk Saving and Loading code in Chunk Class
Chunk Generating, Saving and Loading code in World Class
Making a Jetpack
Summarized Bullet Points:
-
Jetpack is pretty simple, is literally applying an upwards acceleration to player.
-
Jetpack has a fuel gauge, when player is out of fuel, stops applying acceleration. Using the jetpack expends fuel.
-
Jetpack recovers fuel slowly while on the ground, after around two seconds.
​
Making this is pretty simple. So first to keep in mind is that gravity is always being applied, though the vertical velocity is getting canceled every time the player has his or her feet on the ground. So to make a jetpack, the acceleration it gives needs to be stronger than the force of gravity. To simplify the math, just add the acceleration of gravity to the acceleration of the jetpack whenever the jetpack is active. After that the math for actually make it work becomes the exact same as what was already being done converting the gravity into a velocity, and then into a positional translation.
However, before applying the jetpack's force, there should be a delay of some kind, since in this case, the player can jump. Presently this is set to around 0.5 seconds after jumping off the ground that the jetpack's acceleration can start to be applied. As the player uses the jetpack, it burns through it's fuel at a set amount per second. The player can regain the fuel by remaining on the ground a while, and then it will slowly regenerate. The jetpack in this game is set up to be fairly gentle and also slow to both burn through it's fuel and to recover fuel. For a more action oriented experience, it would be better to set the fuel burn and recovery rates higher, as well as put more acceleration behind the jetpack. Similar concepts to the jetpack can be applied for midair dashing, or other such things, as essentially what your doing is launching the character around.
All Code in the JetPack class
Walking Update method from the Player class
Placing and Removing Blocks
-
Raycasting is used to determine what block is in front of the player.
-
Presently mine runs on regular raycasting out a set distance (checks what block in on a regular interval).
-
An optimization would be to get Amanatides Woo Raycasting to work in a 3D space.
-
-
Based on the player's present selection, an associated block type is placed down in front of the player when right click is pressed.
-
One left click held down, the block will start to display a destruction animation over a particular period of time, at the end of which the block will be destroyed if left click is held down the whole time
-
Whenever a player places or removes a block, the chunk is labeled as dirty, and the lighting for it will be updated.
General Update from Player Class.
Important because it shows how selection of block type is handled
World Update and Chunk update related code in the World class
Chunk Lighting Update related code in the Chunk class